Author |
Message
|
Admin Site Admin
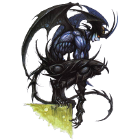
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [782] - posted: 2011-11-21 19:11:13 by the looks of it, this must be a bug in your plugin.
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [783] - posted: 2011-11-21 19:13:07 ok , here is the full code of plugin
Code:plugins off;
plugins emit;
program WIN32DLL 'Masm PLUGIN';
#include 'zirplug/framework.zir';
#include 'zirutils.zir';
//////////////////////////////
const strLoaded = 'Masm plugin version 0.01 has loaded! ';
function H_strlen(char* str) {
Eax = str;
while(char[eax] != 0) {
Eax++;
}
sub eax, str
}
function H_strcpy(Dword dst,src)
{
uses edx ebx;
Eax = dst;
Edx = src;
push Eax;
BL = [Edx];
while ( BL != 0)
{
[Eax] = BL;
Eax++; Edx++;
BL = [Edx];
}
[Eax] = 0;
pop Eax;
}
function H_strcat(Dword dst,src)
{
uses edx ebx;
Eax = dst;
Edx = src;
push Eax;
while (byte [Eax] != 0) { Eax++; }
while (byte [Edx] != 0)
{
BL = [Edx];
[Eax] = BL;
Edx++; Eax++;
}
[Eax] = 0;
pop Eax;
}
inline procedure H_strjoin(;)
{
$temp = 1;
$to = $argc - 1;
$repeat $to:
H_strcat($arg[0],$arg[$temp]);
$temp++;
$end
}
inline procedure GotoLineEnd()
{
eax = Ziron_IsNextLineBreak();
while(!eax)
{
Ziron_GetNextToken(); // remove every things after ';'
eax = Ziron_IsNextLineBreak();
}
}
inline procedure SkipSemiToLineEnd()
{
eax = Ziron_PeekNextToken();
if (eax == zirSemi_Colon)
{
Ziron_GetNextToken(); // remove ';'
GotoLineEnd();
}
}
//////////////////////////////
function event_Data() {
uses edi esi ecx ebx;
char buf[2048];
char var[255];
char right[5100];
char tmp[255];
char dtype[32];
dword indx;
const stp = 16;
boolean Flg = true;
if(Flg) {
// always start with '{'
eax = Ziron_ExpectNextToken(zirBraceOpen);
if (eax == -1) {
Ziron_FatalError('Expected { symbol');
return true;
}
repeat {
// found '}' so let's finished
eax = Ziron_PeekNextToken();
if (eax == zirBraceClose) {
Ziron_GetNextToken();
break;
}
buf=0;
right=0;
indx = 0;
// get the variable name and hold it in Var
eax = Ziron_ExpectNextToken(zirIdent);
if (eax == -1) {
Ziron_FatalError('Expected Variable Name');
return true;
}
H_strcpy(@var, Ziron_GetStringValue());
// get DB or DW or DD or DQ
Ziron_GetNextToken();
boolean _db, _dw, _dd, _dq;
_db = strCmp('db',strLower(Ziron_GetStringValue()));
if(_db)
{
// found DB
H_strcpy(@dtype,'byte ');
}
_dw = strCmp('dw',strLower(Ziron_GetStringValue()));
if(_dw)
{
// found DW
H_strcpy(@dtype,'word ');
}
_dd = strCmp('dd',strLower(Ziron_GetStringValue()));
if(_dw)
{
// found DD
H_strcpy(@dtype,'DWord ');
}
_dd = strCmp('dq',strLower(Ziron_GetStringValue()));
if(_dw)
{
// found DQ
H_strcpy(@dtype,'qword ');
}
if ( (_db) or (_dw) or (_dd) or (_dq) )
{
@digit:
// get whatever after DB
eax = Ziron_GetNextToken();
// var DB ?
if (eax == zirQuestionSym) {
H_strjoin(@buf, @dtype , @var, ' = ' , '0;' );
Ziron_Exec(@buf);
continue;
}
case (eax) {
// var DB 0x0f
state zirHEXADECIMAL:
edx = indx; imul edx, stp; eax = @right; edx += eax;
H_strcpy(edx,'0x');
H_strcat(edx,Ziron_GetStringValue());
break;
// var Db 1
state zirNUMBER:
edx = indx; imul edx, stp; eax = @right; edx += eax;
H_strcpy(edx,Ziron_GetStringValue());
break;
// str DB 'hello',0
state zirConstString:
char str1[2048];
H_strcpy(@str1,Ziron_GetStringValue());
ecx = H_strlen(@str1);
while(ecx > 0)
{
esi = @str1;
edx = indx; bl = [esi+edx]; imul edx, stp; eax = @right; edx += eax;
char[edx] = ord('"');
char[edx+1] = bl;
char[edx+2] = ord('"');
char[edx+3] = 0;
indx++;
ecx--;
}
indx--;
break;
default:
Ziron_FatalError('Expected number');
return true;
}
eax = Ziron_PeekNextToken();
// check for ','
// var db 0 , 0 , 0 , 1
if (eax == zirCOMMA) {
Ziron_GetNextToken(); // remove ','
indx++;
goto @digit;
}
// check for ';'
// var DB 0x0f ; Declare a byte
SkipSemiToLineEnd();
// bytes DB 10 DUP(0)
eax = strCmp('dup',strLower(Ziron_GetStringValue()));
if (eax)
{
Ziron_GetNextToken(); // remove 'DUP'
Ziron_GetNextToken(); // remove '('
Ziron_GetNextToken(); // remove '0'
char v[10];
H_strcpy(@v,Ziron_GetStringValue());
Ziron_GetNextToken(); // remove ')'
ecx = strVal(@right);
while(ecx > 0)
{
edx = indx; imul edx, stp; eax = @right; edx += eax;
H_strcpy(edx,@v);
indx++;
ecx--;
}
indx--;
// check for ';'
SkipSemiToLineEnd();
}
//
buf = 0;
if (indx == 0) {
H_strjoin(@buf, @dtype , @var, ' = ' , @right , ';' );
Ziron_ShowMessage(@buf);
Ziron_Exec(@buf);
}
else
{
H_strcat(@buf, @dtype );
edi = xor;
while (edi <= indx)
{
IntToStr(edi, @tmp);
edx = edi; imul edx, stp; eax = @right; edx += eax;
H_strjoin(@buf, @var, '[' , @tmp ,']=' , edx , '; ' );
edi++;
}
Ziron_ShowMessage(@buf);
Ziron_Exec(@buf);
}
}
else
{
// there is no DB or DW or DD or DQ so fire error
Ziron_FatalError('Expected DB or DW or DD or DQ symple');
return true;
}
};
return true;
} else {
return false; // let Ziron handle the line
}
}
procedure FileEntry(DWord handle; char* strCode) {
eax = strOffset(strCode, '.DATA');
while (char[eax] <> 0) {
char[eax] = "_";
eax = strOffset(eax, '.DATA');
}
eax = strOffset(strCode, '.CODE');
while (char[eax] <> 0) {
char[eax] = "_";
eax = strOffset(eax, '.CODE');
}
}
function InitPlugin(pointer pGetF) {
Ziron_LoadAll(pGetF); //use framework utility function to load all
//register our keyword
Ziron_RegisterKeyword('_Data', @event_Data);
return strLoaded;
}
entry function DLLMain(DWord iDLL; DWord iReason; Pointer iResult) {
if (iReason == DLL_PROCESS_ATTACH) {
return true;
}
return false;
}
exports InitPlugin, FileEntry;
http://www.freewebs.com/ogremagic/index.htm |
Admin Site Admin
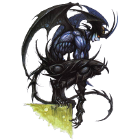
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [784] - posted: 2011-11-21 19:26:53 your bug is here:
Code: _dd = strCmp('dd',strLower(Ziron_GetStringValue()));
if(_dw)
{
// found DD
H_strcpy(@dtype,'DWord ');
}
_dd = strCmp('dq',strLower(Ziron_GetStringValue()));
if(_dw)
{
// found DQ
H_strcpy(@dtype,'qword ');
}
you do the _dd check twice, anyways here is an optimised version:
Code:function event_Data() {
uses edi esi ecx ebx;
char buf[2048];
char var[255];
char right[5100];
char tmp[255];
char dtype[32];
dword indx;
const stp = 16;
boolean Flg = true;
if(Flg) {
// always start with '{'
eax = Ziron_ExpectNextToken(zirBraceOpen);
if (eax == -1) {
Ziron_FatalError('Expected { symbol');
return true;
}
repeat {
// found '}' so let's finished
eax = Ziron_PeekNextToken();
if (eax == zirBraceClose) {
Ziron_GetNextToken();
break;
}
buf=0;
right=0;
indx = 0;
// get the variable name and hold it in Var
eax = Ziron_ExpectNextToken(zirIdent);
if (eax == -1) {
Ziron_FatalError('Expected Variable Name');
return true;
}
H_strcpy(@var, Ziron_GetStringValue());
// get DB or DW or DD or DQ
Ziron_GetNextToken();
boolean _db, _dw, _dd, _dq;
char* strTemp = strLower(Ziron_GetStringValue());
_db = strCmp('db',strTemp);
if (_db) {
H_strcpy(@dtype,'byte '); // found DB
} else {
_dw = strCmp('dw',strTemp); //
if (_dw) {
H_strcpy(@dtype,'word '); // found DW
} else {
_dd = strCmp('dd', strTemp);
if (_dd) {
H_strcpy(@dtype,'DWord '); // found DD
} else {
_dq = strCmp('dq', strTemp);
if (_dq) {
H_strcpy(@dtype,'qword '); // found DQ
} else {
// there is no DB or DW or DD or DQ so fire error
Ziron_FatalError('Expected DB or DW or DD or DQ symple');
return true;
}
}
}
}
@digit:
// get whatever after DB
eax = Ziron_GetNextToken();
// var DB ?
if (eax == zirQuestionSym) {
H_strjoin(@buf, @dtype , @var, ' = ' , '0;' );
Ziron_Exec(@buf);
continue;
}
case (eax) {
// var DB 0x0f
state zirHEXADECIMAL:
edx = indx; imul edx, stp; eax = @right; edx += eax;
H_strcpy(edx,'0x');
H_strcat(edx,Ziron_GetStringValue());
break;
// var Db 1
state zirNUMBER:
edx = indx; imul edx, stp; eax = @right; edx += eax;
H_strcpy(edx,Ziron_GetStringValue());
break;
// str DB 'hello',0
state zirConstString:
char str1[2048];
H_strcpy(@str1,Ziron_GetStringValue());
ecx = H_strlen(@str1);
while(ecx > 0)
{
esi = @str1;
edx = indx; bl = [esi+edx]; imul edx, stp; eax = @right; edx += eax;
char[edx] = ord('"');
char[edx+1] = bl;
char[edx+2] = ord('"');
char[edx+3] = 0;
indx++;
ecx--;
}
indx--;
break;
default:
Ziron_FatalError('Expected number');
return true;
}
eax = Ziron_PeekNextToken();
// check for ','
// var db 0 , 0 , 0 , 1
if (eax == zirCOMMA) {
Ziron_GetNextToken(); // remove ','
indx++;
goto @digit;
}
// check for ';'
// var DB 0x0f ; Declare a byte
SkipSemiToLineEnd();
// bytes DB 10 DUP(0)
eax = strCmp('dup',strLower(Ziron_GetStringValue()));
if (eax)
{
Ziron_GetNextToken(); // remove 'DUP'
Ziron_GetNextToken(); // remove '('
Ziron_GetNextToken(); // remove '0'
char v[10];
H_strcpy(@v,Ziron_GetStringValue());
Ziron_GetNextToken(); // remove ')'
ecx = strVal(@right);
while(ecx > 0)
{
edx = indx; imul edx, stp; eax = @right; edx += eax;
H_strcpy(edx,@v);
indx++;
ecx--;
}
indx--;
// check for ';'
SkipSemiToLineEnd();
}
//
buf = 0;
if (indx == 0) {
H_strjoin(@buf, @dtype , @var, ' = ' , @right , ';' );
Ziron_ShowMessage(@buf);
Ziron_Exec(@buf);
}
else
{
H_strcat(@buf, @dtype );
edi = xor;
while (edi <= indx)
{
IntToStr(edi, @tmp);
edx = edi; imul edx, stp; eax = @right; edx += eax;
H_strjoin(@buf, @var, '[' , @tmp ,']=' , edx , '; ' );
edi++;
}
Ziron_ShowMessage(@buf);
Ziron_Exec(@buf);
}
};
return true;
} else {
return false; // let Ziron handle the line
}
}
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Admin Site Admin
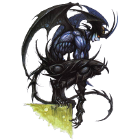
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [785] - posted: 2011-11-21 19:31:30 btw i would recommend to not do the strCmp for db, dw etc, and use the masm include file, that way you can just expect a data type and it wont matter, it would also allow people to use default ziron types with your masm plugin.
e.g. allowing:
Code:
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [786] - posted: 2011-11-21 19:32:36
ok , thanks for the optimization , i will remove qword until ziorn will support it.
http://www.freewebs.com/ogremagic/index.htm |
Admin Site Admin
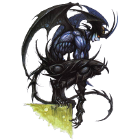
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [787] - posted: 2011-11-21 19:34:59 i'm just looking at adding it now to allow for int64 within 32bit environment. 
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [792] - posted: 2011-11-22 19:47:27
ok , masm plugin now understand this
Code:program WIN32CUI 'Masm Syntax test';
#include 'console.zir';
.DATA
{
var DB 0x0f ; Declare a byte, referred to as location var, containing the value 0x0f.
vv Db 1
ar1 DB ?
ar2 DB 0
ml db 0 , 0 , 0 , 1
bytes DB 10 DUP(0) ; Declare 10 bytes starting at location bytes.
str1 DB 'hello',0
Wvar dw 10
Dvar dd 20
//Qvar dq 30 // will be allowed later
varname dword 1342 , 10
vwrd word 7
vbyte byte 0x40
vchar char 'welcome',13,10,0
}
wait_key(nil);
ExitProcess(0);
any suggestion will be welcome.
http://www.freewebs.com/ogremagic/index.htm |
Admin Site Admin
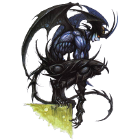
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [793] - posted: 2011-11-22 19:50:20 does it also support single, double and extended ?
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |