Author |
Message
|
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [727] PlugIn Example in C - posted: 2011-11-18 10:24:13
Hi
i have converted Framework.zir to framework.h , also i made a test plugin in C
i have used Pelles C to compile this project
downlod it here http://spoilerspace.com//bcxdx/Ziron/zirPlugin.zip
Code:/****************************************************************************
* *
* File : dllmain.c *
* *
* Purpose : Generic Win32 Dynamic Link Library (DLL). *
* *
* History : Date Reason *
* 00/00/00 Created *
* *
****************************************************************************/
#define WIN32_LEAN_AND_MEAN /* speed up */
#include <windows.h>
#include "framework.h"
#include "ZIRPLUGIN.h"
//////////////////////////////
char* strLoaded = "My plugin has loaded!";
//////////////////////////////
//
// event_Emit()
// return false to allow the assembler to process this keyword internally.
// return true to tell the assembler this keyword has been processed.
//
BOOL event_Emit(void)
{
Ziron_ShowMessage("hellow from plugin written with C.");
return TRUE;
}
char* InitPlugin(void* pGetF)
{
Ziron_LoadAll(pGetF); //use framework utility function to load all
//register our keyword
Ziron_RegisterKeyword("emit", event_Emit);
return strLoaded;
}
/****************************************************************************
* *
* Function: DllMain *
* *
* Purpose : DLL entry and exit procedure. *
* *
* History : Date Reason *
* 00/00/00 Created *
* *
****************************************************************************/
BOOL APIENTRY DllMain(HINSTANCE hInstDLL, DWORD fdwReason, LPVOID lpvReserved)
{
/* Return success */
return TRUE;
}
http://www.freewebs.com/ogremagic/index.htm |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [736] - posted: 2011-11-19 17:32:53 ok , i have continue developing in Ziron again , so here is what i made till now.
Code:plugins off;
plugins emit;
program WIN32DLL 'Masm PLUGIN';
#include 'zirplug/framework.zir';
#include 'zirutils.zir';
//////////////////////////////
const strLoaded = 'Masm plugin has loaded!';
function H_strlen(char* str) {
Eax = str;
while(char[eax] != 0) {
Eax++;
}
sub eax, str
}
function H_strcpy(Dword dst,src)
{
uses edx ebx;
Eax = dst;
Edx = src;
push Eax;
BL = [Edx];
while ( BL != 0)
{
[Eax] = BL;
Eax++; Edx++;
BL = [Edx];
}
[Eax] = 0;
pop Eax;
}
function H_strcat(Dword dst,src)
{
uses edx ebx;
Eax = dst;
Edx = src;
push Eax;
while (byte [Eax] != 0) { Eax++; }
while (byte [Edx] != 0)
{
BL = [Edx];
[Eax] = BL;
Edx++; Eax++;
}
[Eax] = 0;
pop Eax;
}
inline procedure H_strjoin(;)
{
$temp = 1;
$to = $argc - 1;
$repeat $to:
H_strcat($arg[0],$arg[$temp]);
$temp++;
$end
}
inline procedure GotoLineEnd()
{
eax = Ziron_IsNextLineBreak();
while(!eax)
{
Ziron_GetNextToken(); // remove every things after ';'
eax = Ziron_IsNextLineBreak();
}
}
inline procedure SkipSemiToLineEnd()
{
eax = Ziron_PeekNextToken();
if (eax == zirSemi_Colon)
{
Ziron_GetNextToken(); // remove ';'
GotoLineEnd();
}
}
//////////////////////////////
function event_Data() {
uses edi esi ecx ebx;
char buf[2048];
char var[255];
char right[5100];
char tmp[255];
dword indx;
const stp = 16;
boolean Flg = true;
if(Flg) {
// always start with '{'
eax = Ziron_ExpectNextToken(zirBraceOpen);
if (eax == -1) {
Ziron_FatalError('Expected { symbol');
return true;
}
repeat {
// found '}' so let's finished
eax = Ziron_PeekNextToken();
if (eax == zirBraceClose) {
Ziron_GetNextToken();
break;
}
buf=0;
right=0;
indx = 0;
// get the variable name and hold it in Var
eax = Ziron_ExpectNextToken(zirIdent);
if (eax == -1) {
Ziron_FatalError('Expected Variable Name');
return true;
}
H_strcpy(@var, Ziron_GetStringValue());
http://www.freewebs.com/ogremagic/index.htm |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [737] - posted: 2011-11-19 17:33:36 Code: // get DB or DW or DD or DQ
eax = Ziron_ExpectNextToken(zirIdent);
if (eax == -1) {
Ziron_FatalError('Expected Variable Name');
return true;
}
eax = strCmp('db',strLower(Ziron_GetStringValue()));
// found DB
if (eax)
{
@digit:
// get whatever after DB
eax = Ziron_GetNextToken();
if (eax == zirQuestionSym) {
H_strjoin(@buf, 'byte ' , @var, ' = ' , '0;' );
Ziron_Exec(@buf);
continue;
}
case (eax) {
state zirHEXADECIMAL:
edx = indx; imul edx, stp; eax = @right; edx += eax;
H_strcpy(edx,'0x');
H_strcat(edx,Ziron_GetStringValue());
break;
state zirNUMBER:
edx = indx; imul edx, stp; eax = @right; edx += eax;
H_strcpy(edx,Ziron_GetStringValue());
break;
state zirConstString:
Ziron_ShowMessage('found string');
char str1[2048];
H_strcpy(@str1,Ziron_GetStringValue());
ecx = H_strlen(@str1);
while(ecx > 0)
{
esi = @str1;
edx = indx; bl = [esi+edx]; imul edx, stp; eax = @right; edx += eax;
char[edx] = ord('"');
char[edx+1] = bl;
char[edx+2] = ord('"');
char[edx+3] = 0;
indx++;
ecx--;
}
indx--;
break;
default:
Ziron_FatalError('Expected number');
return true;
}
eax = Ziron_PeekNextToken();
// check for ','
if (eax == zirCOMMA) {
Ziron_GetNextToken(); // remove ','
indx++;
goto @digit;
}
// check for ';'
SkipSemiToLineEnd();
eax = strCmp('dup',strLower(Ziron_GetStringValue()));
if (eax)
{
Ziron_GetNextToken(); // remove 'DUP'
Ziron_ShowMessage('found DUP');
Ziron_GetNextToken(); // remove '('
Ziron_GetNextToken(); // remove '0'
Ziron_GetNextToken(); // remove ')'
// check for ';'
SkipSemiToLineEnd();
}
buf = 0;
if (indx == 0) {
H_strjoin(@buf, 'byte ' , @var, ' = ' , @right , ';' );
Ziron_ShowMessage(@buf);
Ziron_Exec(@buf);
}
else
{
H_strcat(@buf, 'byte ' );
edi = xor;
while (edi <= indx)
{
IntToStr(edi, @tmp);
edx = edi; imul edx, stp; eax = @right; edx += eax;
H_strjoin(@buf, @var, '[' , @tmp ,']=' , edx , '; ' );
edi++;
}
Ziron_ShowMessage(@buf);
Ziron_Exec(@buf);
}
}
};
return true;
} else {
return false; // let Ziron handle the line
}
}
procedure FileEntry(DWord handle; char* strCode) {
eax = strOffset(strCode, '.DATA');
while (char[eax] <> 0) {
char[eax] = "_";
eax = strOffset(eax, '.DATA');
}
eax = strOffset(strCode, '.CODE');
while (char[eax] <> 0) {
char[eax] = "_";
eax = strOffset(eax, '.CODE');
}
}
function InitPlugin(pointer pGetF) {
Ziron_LoadAll(pGetF); //use framework utility function to load all
//register our keyword
Ziron_RegisterKeyword('_Data', @event_Data);
return strLoaded;
}
entry function DLLMain(DWord iDLL; DWord iReason; Pointer iResult) {
if (iReason == DLL_PROCESS_ATTACH) {
return true;
}
return false;
}
exports InitPlugin, FileEntry;
http://www.freewebs.com/ogremagic/index.htm |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [739] - posted: 2011-11-19 17:35:09 here is the test program
Code:program WIN32CUI 'Masm Syntax test';
#include 'console.zir';
.DATA
{
var DB 0x0f ; Declare a byte, referred to as location var, containing the value 0x0f.
vv Db 1
ar1 DB ?
ar2 DB 0
ml db 0 , 0 , 0 , 1
bytes DB 10 DUP(0) ; Declare 10 uninitialized bytes starting at location bytes.
str1 DB 'hello',0
}
eax = @ml;
Ecx = [eax]
print('var = ', Ecx);
wait_key(nil);
ExitProcess(0);
so any help or suggestion will be welcome.
http://www.freewebs.com/ogremagic/index.htm |
Admin Site Admin
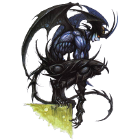
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [740] - posted: 2011-11-19 17:36:11 looking good, later today i will extend the maximum posting size so any further posts can be in bigger chunks 
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [769] - posted: 2011-11-21 17:02:43 Ziron points me to wrong line which made the error
here is the error log file
Code:[142,28]: Expected ; or >> or << or += or -= or = or ( or as or ++ or -- but found ) in [masm.zir]
Code:
Ziron_Exec(@buf);
here is the code ,BTW you have mentioned that you will allow forum accept long post!!!!!!!!!!
Code:plugins off;
plugins emit;
program WIN32DLL 'Masm PLUGIN';
#include 'zirplug/framework.zir';
#include 'zirutils.zir';
//////////////////////////////
const strLoaded = 'Masm plugin has loaded!';
function H_strlen(char* str) {
Eax = str;
while(char[eax] != 0) {
Eax++;
}
sub eax, str
}
function H_strcpy(Dword dst,src)
{
uses edx ebx;
Eax = dst;
Edx = src;
push Eax;
BL = [Edx];
while ( BL != 0)
{
[Eax] = BL;
Eax++; Edx++;
BL = [Edx];
}
[Eax] = 0;
pop Eax;
}
function H_strcat(Dword dst,src)
{
uses edx ebx;
Eax = dst;
Edx = src;
push Eax;
while (byte [Eax] != 0) { Eax++; }
while (byte [Edx] != 0)
{
BL = [Edx];
[Eax] = BL;
Edx++; Eax++;
}
[Eax] = 0;
pop Eax;
}
inline procedure H_strjoin(;)
{
$temp = 1;
$to = $argc - 1;
$repeat $to:
H_strcat($arg[0],$arg[$temp]);
$temp++;
$end
}
http://www.freewebs.com/ogremagic/index.htm |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [770] - posted: 2011-11-21 17:04:42 Code:inline procedure GotoLineEnd()
{
eax = Ziron_IsNextLineBreak();
while(!eax)
{
Ziron_GetNextToken(); // remove every things after ';'
eax = Ziron_IsNextLineBreak();
}
}
inline procedure SkipSemiToLineEnd()
{
eax = Ziron_PeekNextToken();
if (eax == zirSemi_Colon)
{
Ziron_GetNextToken(); // remove ';'
GotoLineEnd();
}
}
//////////////////////////////
function event_Data() {
uses edi esi ecx ebx;
char buf[2048];
char var[255];
char right[5100];
char tmp[255];
char dtype[32];
dword indx;
const stp = 16;
boolean Flg = true;
if(Flg) {
// always start with '{'
eax = Ziron_ExpectNextToken(zirBraceOpen);
if (eax == -1) {
Ziron_FatalError('Expected { symbol');
return true;
}
repeat {
// found '}' so let's finished
eax = Ziron_PeekNextToken();
if (eax == zirBraceClose) {
Ziron_GetNextToken();
break;
}
buf=0;
right=0;
indx = 0;
// get the variable name and hold it in Var
eax = Ziron_ExpectNextToken(zirIdent);
if (eax == -1) {
Ziron_FatalError('Expected Variable Name');
return true;
}
H_strcpy(@var, Ziron_GetStringValue());
// get DB or DW or DD or DQ
eax = Ziron_ExpectNextToken(zirIdent);
if (eax == -1) {
Ziron_FatalError('Expected DB or DW or DD or DQ symple');
return true;
}
boolean _db, _dw;
_db = strCmp('db',strLower(Ziron_GetStringValue()));
if(_db)
{
// found DB
H_strcpy(@dtype,'byte ');
goto @digit;
}
_dw = strCmp('dw',strLower(Ziron_GetStringValue()));
if(_dw)
{
// found DW
H_strcpy(@dtype,'word ');
goto @digit;
}
Ziron_FatalError('Expected DB or DW or DD or DQ symple');
return true;
http://www.freewebs.com/ogremagic/index.htm |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [771] - posted: 2011-11-21 17:06:18 Code: // if (_db == true or _dw == true)
// {
@digit:
// get whatever after DB
eax = Ziron_GetNextToken();
// var DB ?
if (eax == zirQuestionSym) {
H_strjoin(@buf, @dtype , @var, ' = ' , '0;' );
Ziron_Exec(@buf);
continue;
}
case (eax) {
// var DB 0x0f
state zirHEXADECIMAL:
edx = indx; imul edx, stp; eax = @right; edx += eax;
H_strcpy(edx,'0x');
H_strcat(edx,Ziron_GetStringValue());
break;
// var Db 1
state zirNUMBER:
edx = indx; imul edx, stp; eax = @right; edx += eax;
H_strcpy(edx,Ziron_GetStringValue());
break;
// str DB 'hello',0
state zirConstString:
char str1[2048];
H_strcpy(@str1,Ziron_GetStringValue());
ecx = H_strlen(@str1);
while(ecx > 0)
{
esi = @str1;
edx = indx; bl = [esi+edx]; imul edx, stp; eax = @right; edx += eax;
char[edx] = ord('"');
char[edx+1] = bl;
char[edx+2] = ord('"');
char[edx+3] = 0;
indx++;
ecx--;
}
indx--;
break;
default:
Ziron_FatalError('Expected number');
return true;
}
eax = Ziron_PeekNextToken();
// check for ','
// var db 0 , 0 , 0 , 1
if (eax == zirCOMMA) {
Ziron_GetNextToken(); // remove ','
indx++;
goto @digit;
}
// check for ';'
// var DB 0x0f ; Declare a byte
SkipSemiToLineEnd();
// bytes DB 10 DUP(0)
eax = strCmp('dup',strLower(Ziron_GetStringValue()));
if (eax)
{
Ziron_GetNextToken(); // remove 'DUP'
Ziron_GetNextToken(); // remove '('
Ziron_GetNextToken(); // remove '0'
char v[8];
H_strcpy(@v,Ziron_GetStringValue());
Ziron_GetNextToken(); // remove ')'
ecx = strVal(@right);
while(ecx > 0)
{
edx = indx; imul edx, stp; eax = @right; edx += eax;
H_strcpy(edx,@v);
indx++;
ecx--;
}
indx--;
// check for ';'
SkipSemiToLineEnd();
}
//
buf = 0;
if (indx == 0) {
H_strjoin(@buf, @dtype , @var, ' = ' , @right , ';' );
Ziron_ShowMessage(@buf);
Ziron_Exec(@buf);
}
else
{
H_strcat(@buf, @dtype );
edi = xor;
while (edi <= indx)
{
IntToStr(edi, @tmp);
edx = edi; imul edx, stp; eax = @right; edx += eax;
H_strjoin(@buf, @var, '[' , @tmp ,']=' , edx , '; ' );
edi++;
}
Ziron_ShowMessage(@buf);
Ziron_Exec(@buf);
}
// }
};
return true;
} else {
return false; // let Ziron handle the line
}
}
procedure FileEntry(DWord handle; char* strCode) {
eax = strOffset(strCode, '.DATA');
while (char[eax] <> 0) {
char[eax] = "_";
eax = strOffset(eax, '.DATA');
}
eax = strOffset(strCode, '.CODE');
while (char[eax] <> 0) {
char[eax] = "_";
eax = strOffset(eax, '.CODE');
}
}
function InitPlugin(pointer pGetF) {
Ziron_LoadAll(pGetF); //use framework utility function to load all
//register our keyword
Ziron_RegisterKeyword('_Data', @event_Data);
return strLoaded;
}
entry function DLLMain(DWord iDLL; DWord iReason; Pointer iResult) {
if (iReason == DLL_PROCESS_ATTACH) {
return true;
}
return false;
}
exports InitPlugin, FileEntry;
it is hard to post the file in 3 parts ,so please allow long post.
http://www.freewebs.com/ogremagic/index.htm |