Author |
Message
|
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [554] - posted: 2011-11-10 07:53:10
ok ihave fixed it , here is the correct code
Code:/***************************************
* Sphinx C-- *
* *
* strcmp demo By Emil Halim *
* 23 / 9 / 2011 *
***************************************/
#pragma option w32 //create Windows GUI EXE.
#pragma option OBJ //create OBJ file
#pragma option OS //speed optimization
#pragma option J0 //no startup code.
#include <Windows.h>
#pragma option ia // allow inline asm
#pragma option LST
extern cdecl _printf();
#define printf _printf
/***************
s > t >>> > 0
s = t >>> = 0
s < t >>> < 0
***************/
int strcmp1(char *s, char *t) // pure C code
{
for( ;byte *s == byte *t; s++, t++)
if (byte *s == '\0' && byte *t == '\0') return 0;
return *s - *t;
}
int fastcall strcmp2(ESI,EDI) // mixed asm & C
{
AL = DSBYTE[ESI];
while(AL==DSBYTE[EDI])
{
if(AL==0) && (DSBYTE[EDI] ==0) return 0;
ESI++; EDI++;
AL = DSBYTE[ESI];
}
return DSBYTE[ESI] - DSBYTE[EDI];
}
char* testStr1 = "SPHINX C-- is so easy (an intermediate position between Assembler and C)";
char* testStr2 = "SPHINX C-- is so easy (an intermediate position between Assembler and C) ";
main()
{
_start:
printf("string length is %d\n",strcmp1( testStr1 , testStr2 ));
printf("string length is %d\n",strcmp2( testStr1 , testStr2 ));
MessageBox(0,"","",0);
}
http://www.freewebs.com/ogremagic/index.htm |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [555] - posted: 2011-11-10 09:30:13
when try convert it to Ziron got that error
“ | [196,26]: Expected register or data type or variable or local variable or local parameter or constant or number or constant string or - but found [edi] in [Helper.zir]
Code:
while(AL == char[EDI]) |
the code
Code:function H_strcmp(Dword str1,str2)
{
ESI = str1; EDI = str2;
AL = char[ESI];
while(AL == char[EDI])
{
if(AL == 0 && char[EDI] ==0){ return 0; }
ESI++; EDI++;
AL = char[ESI];
}
xor Eax,Eax
al = = char[ESI];
al -= char[EDI];
}
so it seems While did not accept parameters just like if statement.
http://www.freewebs.com/ogremagic/index.htm |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [556] - posted: 2011-11-10 09:36:11
also why Ziron do not allow this expression
Code:if(strCmp(Ziron_GetVarTypeString(eax),'int32') == 0)
http://www.freewebs.com/ogremagic/index.htm |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [557] - posted: 2011-11-10 10:23:33
here is what i done , oh.... the H_strjoin gives access violation
Code:function event_Let() {
uses edi;
char buf[2048];
char var[255];
char str1[255];
Dword Len;
boolean StringFlg;
// get the variable name and hold it in Var
eax = Ziron_GetNextToken();
case (eax) {
state zironVar:
state zironLocalVar:
break;
default:
Ziron_FatalError('Expected Variable Name');
return true;
}
H_strcpy(@var,Ziron_GetStringValue());
StringFlg = strCmp(Ziron_GetVarTypeString(Ziron_GetID()),'char');
// check for'=' symbol
eax = Ziron_ExpectNextToken(zirAssignment);
if (eax == -1) {
Ziron_FatalError('Expected = symbol');
return true;
}
// start pares if the var type is string
if(StringFlg)
{
// here we start getting what after '='
Ziron_GetNextToken();
H_strcpy(@str1,Ziron_GetStringValue());
eax = Ziron_IsNextLineBreak();
if (!eax) {
Ziron_GetNextToken(); // remove ';'
}
buf=0;
H_strjoin(@buf, 'H_strcpy(@' , @var, ', \'' , str1, '\');' ); // access violation here
Ziron_ShowMessage(@buf);
return true;
}
else
{
return false; // let Ziron handle the line
}
}
http://www.freewebs.com/ogremagic/index.htm |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [558] - posted: 2011-11-10 10:42:39
oh , my mistake , forgot '@' ,any way here is a working Let code
Code:function event_Let() {
uses edi;
char buf[2048];
char var[255];
char str1[255];
Dword Len;
boolean StringFlg;
// get the variable name and hold it in Var
eax = Ziron_GetNextToken();
case (eax) {
state zironVar:
state zironLocalVar:
break;
default:
Ziron_FatalError('Expected Variable Name');
return true;
}
H_strcpy(@var,Ziron_GetStringValue());
StringFlg = strCmp(Ziron_GetVarTypeString(Ziron_GetID()),'char');
// check for'=' symbol
eax = Ziron_ExpectNextToken(zirAssignment);
if (eax == -1) {
Ziron_FatalError('Expected = symbol');
return true;
}
// start pares if the var type is string
if(StringFlg)
{
// here we start getting what after '='
Ziron_GetNextToken();
H_strcpy(@str1,Ziron_GetStringValue());
eax = Ziron_IsNextLineBreak();
if (!eax) {
Ziron_GetNextToken(); // remove ';'
}
buf=0;
H_strjoin(@buf, 'H_strcpy(@' , @var, ', \'' , @str1, '\');' );
Ziron_ShowMessage(@buf);
Len = H_strlen(@buf);
eax = Ziron_Execute(@buf,Len);
if (eax == false) {
Ziron_FatalError('Dim plugin is not compatible with this version of Ziron');
return true;
}
return true;
}
else
{
return false; // let Ziron handle the line
}
}
function InitPlugin(pointer pGetF) {
Ziron_LoadAll(pGetF); //use framework utility function to load all
//add H_strcpy function
const sc_code = 'function H_strcpy(Dword dst,src) { Eax = dst; Edx = src; push Eax; BL = [Edx]; while ( BL != 0) { [Eax] = BL; Eax++; Edx++; BL = [Edx]; } [Eax] = 0; pop Eax; }';
Ziron_Execute(@sc_code,H_strlen(@sc_code));
//add some variable type
const MyType = 'type Integer = Int32; type String = char; type Uchar = char; type Schar = sbyte; type USHORT = word;';
Ziron_Execute(@MyType,H_strlen(@MyType));
//register our keyword
Ziron_RegisterKeyword('Dim', @event_Dim);
Ziron_RegisterKeyword('Let', @event_Let);
return strLoaded;
}
as you see , in InitPlugin i have putted H_strcpy implementation , but need to check if it
already implemented before or not
here is a test code
Code:DIM Name$
print('Name = ');
Let Name = '100';
WriteLnEx(@Name,4);
http://www.freewebs.com/ogremagic/index.htm |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [559] - posted: 2011-11-10 11:38:23
I need to add those symbols to enum ZirToken
Code:
http://www.freewebs.com/ogremagic/index.htm |
Emil_halim Ziron Beta Tester

(send private message)
Posts: 639 Topics: 104
Location: Alex, Egypt | [560] - posted: 2011-11-10 12:11:57
it seems there is problem with zirPlusSym
so this code does not work as expected
Code: eax = Ziron_IsNextLineBreak();
if (!eax) {
eax = Ziron_GetNextToken();
case (eax) {
state zirPlusSym:
state zirComma:
Ziron_ShowMessage('found + or ,');
break;
// remove ';' if found
state zirSemi_Colon:
Ziron_ShowMessage('found ;');
break;
default:
Ziron_FatalError('Expected "+" or ";"');
return true;
}
}
test code give this error
“ |
Expected number or hexadecimal or constant string but found wait_key in [testprog.zir]
Code:
wait_key(nil);
|
Code:Dim c$
Let c = 'Emil' + 'halim'
wait_key(nil);
ExitProcess(0);
http://www.freewebs.com/ogremagic/index.htm |
Admin Site Admin
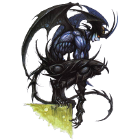
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [561] - posted: 2011-11-10 13:40:08
“ |
when try convert it to Ziron got that error
|
switch the direction, it is a known bug, i need to put more work into the high level comparison code.
Code:
“ |
also why Ziron do not allow this expression
|
calling functions inside of block statements is not valid in Ziron - this is something that i may work on in later versions.
Code:eax = strCmp(Ziron_GetVarTypeString(eax), 'int32');
if (eax == 0)
“ |
as you see , in InitPlugin i have putted H_strcpy implementation , but need to check if it
already implemented before or not
|
this is what un-named function feature will be for, hopefully for next update will be ready.
“ |
I need to add those symbols to enum ZirToken
|
these tokens are detected as identifiers, however, i believe i may add them in the future, so i will add them for next release.
“ |
Let c = 'Emil' + 'halim'
|
the reason you are not detecting the + sym is because ziron already is putting Emil and halim together and removing the plus sym.
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |