Author |
Message
|
Admin Site Admin
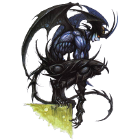
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [1249] - posted: 2012-12-06 23:57:00 I'm back for another release with a few updates and fixes
Code:2012.12.06: Ziron Compiler 1.1.36.1
-added jecxz jump instruction.
-added cmovz reg, reg instruction
-fixed compare reg with hexadecimal.
-added optimization on high level compare of register to 0 or null.
-added break if (...) syntax.
-continue now can accept an offset value.
-added continue if (...) syntax.
This release has an optimization for comparing registers with nil or 0 and also continue and break if statements which can be very useful for optimising your code.
here's a sample function that uses break if
Code:function getFirstChar(char* str; char _c) {
ecx = str;
al = [ecx]
ah = _c;
if (al != 0) {
repeat {
break if (al == ah);
ecx += 1;
al = [ecx];
} until (al == 0);
}
eax = ecx;
eax += 1;
}
check the download page for release 1.1.36.1
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Admin Site Admin
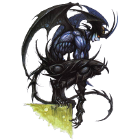
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [1250] - posted: 2012-12-08 16:37:44 Here we go with another quick release, it is noted that if any of your code relies on classes, you should not upgrade to this version, classes are temporarily depreciated as i want to give them an overhaul and maybe add some compatibility with other languages such as c++, but this is another story..
classes are replaced with block functions, the new structure allows you to declare methods inside of a block/struct and then later in your code write the code, this has the advantage of the old style classes at the same time you can now call methods out of order. Here is an example:
Code:program WIN32CUI 'building_blocks';
#include 'ch.zir';
// we will define a useless structure with 2 useless functions
block ZNewBlock {
DWord a;
DWord b;
//here we add the declarations of the functions
function getBase(): Int32;
function getSum(): Int32;
}
//now we need to add the actual code for the functions
function ZNewBlock:getSum() {
//notice that we can now call getBase out of order
eax = this.getBase();
eax += this.a;
eax += this.b
}
function ZNewBlock:getBase() {
eax = 100;
}
/////////////////
ZNewBlock myBlock;
myBlock.a = 5;
myBlock.b = 10;
edx = myBlock.getSum();
print('Result = ', edx, '\r\n');
wait_key();
ExitProcess(0);
one great thing to note about the block methods is that all registers are since the stack is managed using esp so that ebp could be freed for the this object, so if you decide you don't need "this" you can even still use the ebp register, I plan also to add block static methods to save on some space and increase performance hopefully for the follow-up.
the changelog for this version:
Code:2012.12.08: Ziron Compiler 1.1.37.7
-high level assignment from register to nil or 0 will automatically be optimised.
-fixed an out of scope problem with pointer functions.
-fixed += and -= for "this" object.
-added support for methods inside blocks/structs
-please note that classes are depreciated and should not be used as they will be rewritten for
an alternative purpose in a later release.
-fixed single line if's without braces.
-added a new sample "structures"
check the download page 
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Admin Site Admin
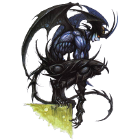
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [1264] - posted: 2012-12-19 15:40:23 Latest version contains a bug preventing some things form working correctly.
Unfortunately the structure of the internal code of Ziron has become very large and unmanageable that some things will not be fixed in this version as i will concentrate on Ziron 2, Ziron 2 will allow for better and faster improvements.
for now download the beta version at
Ziron Beta
ps another sample of blocks with functions
Code:program WIN32CUI 'test';
#include 'ch.zir';
#include 'fileio.zir';
////////
block ZFile {
DWord handle;
function open(char* filename): boolean;
procedure close();
function writeBuffer(char* text): boolean;
function readBuffer(char* buf): boolean;
}
function ZFile:open(char* filename) {
const flag = OPEN_EXISTING;
eax = CreateFileA(filename, GENREADWRITE, 0, 0, flag, FILE_ATTRIBUTE_NORMAL, nil);
this.handle = eax;
if (eax <> nil) {
return true;
}
return false;
}
procedure ZFile:close() {
CloseHandle(this.handle);
}
function ZFile:writeBuffer(char* text) {
DWord bytesWritten;
WriteFile(this.handle, text, strLen(text), @bytesWritten, 0);
}
function ZFile:readBuffer(char* buf; DWord len) {
DWord bytesRead;
ReadFile(this.handle, buf, len, @bytesRead, nil);
return eax;
}
////////
ZFile file;
char buf[512];
/////
file.open('text.txt');
file.writeBuffer('Hello, World!\r\n');
//file.readBuffer(@buf, 512);
file.close();
//print(@buf);
wait_key();
ExitProcess(0);
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Admin Site Admin
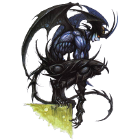
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [1267] - posted: 2012-12-22 15:21:52 A new release to fix a couple bugs and to showcase the new block system a little.
Code:2012.12.22: Ziron Compiler 1.1.38.1
-added thread sample (see samples\threads\threads.zir)
-fixed a problem with pushing and moving @this inside of block functions.
-added block style class ZThread. (see includes\threading.zir)
-added block style class ZFile. (see includes\fileio.zir)
-fixed macro wait_key.
-fixed problem with block functions, temporary fix for compatibility for Ziron 2. (may contain bugs in this fix)
-labels no longer require @ symbol, the label @ symbol is depreciated
check downloads page for download link 
a new sample threads.zir:
Code:program WIN32CUI 'test';
#include 'ch.zir';
#include 'threading.zir';
//////////////
function stdcall myFunc(ZThread* this) {
uses ebx;
ebx = this;
// [ebx+0] = handle
// [ebx+4] = id
// [ebx+8] = active
while ([ebx+8]) {
print('Hello, World from Thread!\r\n');
sleep(100);
}
print('Thread ending!\r\n');
}
ZThread myThread;
eax = myThread.Execute(@myFunc);
if (eax == False) {
print('Error creating thread!\r\n');
}
ebx = 1;
repeat {
print('Hello, World from Main Thread :)\r\n');
ebx += 1;
sleep(200);
} until (ebx > 10);
wait_key('Press any key to abort thread');
myThread.Abort();
//let threads finish, we could do some checking here if we prefere :)
sleep(100);
wait_key();
ExitProcess(0);
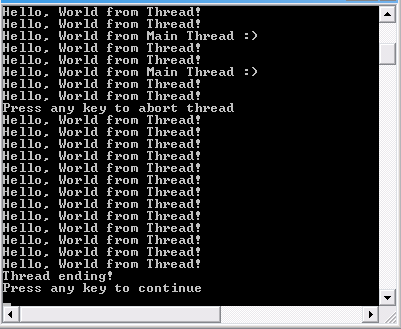
let me know 
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Admin Site Admin
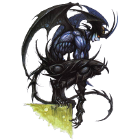
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [1322] - posted: 2013-01-12 15:44:57 The time has come for the first release of 2013... with this first is 2 major improvements... I have rewritten the PECOFF linker and i have also written a MS COFF object builder, they are both in experimental stages so please let me know of any bugs.
Code:2013.01.12: Ziron Compiler 1.2.0.0
-written internal MS COFF builder (experimental)
-rewrote PE coff linker (experimental)
-now possible for imports to have default parameters.
-possible to have floats as default parameter.
-ziron will now push single, double and extended in respect to the parameter type of the called procedure.
-fixed mov opcode mov double var.
-fixed high level assign floats to variables.
-fixed calling functions with - integer and float values (floats are passed as real4.
-changed importing mangled names .... function name = ('name?name@@12')(params ....
Here is an example of building and linking an app with vs link.exe
Code:program MSCOFF 'test';
#include 'user32.zir';
#include 'kernel32.zir';
const strTitle = 'Hello!';
const strMsg = 'Hello, World!';
procedure showMessage(char* msg) {
MessageBoxA(0, msg, strTitle, 0);
}
showMessage(strMsg);
ExitProcess(0);
build with ziron and then linking would be as follows:
Code:link.exe /SUBSYSTEM:windows /ENTRY:entry user32.lib kernel32.lib yourapp.obj
a thing to note is entry is always named "entry".
This will output your exe that will show a simple hello world messagebox.
check the download page 
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Admin Site Admin
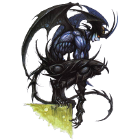
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [1323] - posted: 2013-01-16 18:15:41 Unfortunately the previous release was a failure, due to a small bug caused by an upgrade of my dev machine to windows 8, anyways this has been solved and a couple other things, so i suggest to update ASAP if you are using 1.2.0.0
Code:2013.01.16: Ziron Compiler 1.2.0.6
-optimised linker.
-fixed an internal build problem on windows 8.
-fixed small problem with functions symbols in object files.
-cdecl, stdcall and fastcall are now correctly added with mangled names to object files.
check download page.
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Admin Site Admin
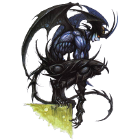
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [1328] - posted: 2013-02-02 19:03:01 A new release with a big fix and a few other additions
Code:2013.02.02: Ziron Compiler 1.2.1.1
-fixed a big error with block functions recieving twice the amount of param count.
-inline macros may now override keywords, but will not allow op style inlines. e.g. inline function add($a, $b)
-objects will now have zircall functions, mangled as so "@_funcname@paramsize"
-ziron will no longer auto pause when error if nopause param is passed, instead will return errorlevel 1
-added compiler param -i "path" for setting another include path.
check download page
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |
Admin Site Admin
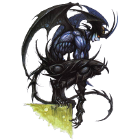
(send private message)
Posts: 933 Topics: 55
Location: OverHertz Studio | [1332] - posted: 2013-02-17 01:32:22 a small update that fixes 2 bugs since the coff linker was rewritten.
Code:2013.02.17: Ziron Compiler 1.2.1.4
-fixed entry offset, was broke since rewrite of coff linker.
-fixed exports, broken also since coff linker rewrite.
Download Ziron
Get free hosting for Ziron related fan-sites and Ziron projects, contact me in private message. |